Disabling Past or Future Dates in Calendar
2 minutes to readDisabling past or future dates in your calendar can be helpful in applications where either past or future is irrelevant (for example, planning itineraries). In this article, we will guide you to disable past or future dates based on another field in a Submission Form. In our example, we will use the context of departure and return dates.
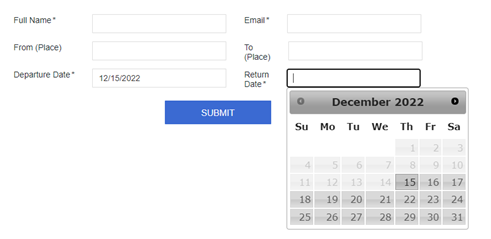
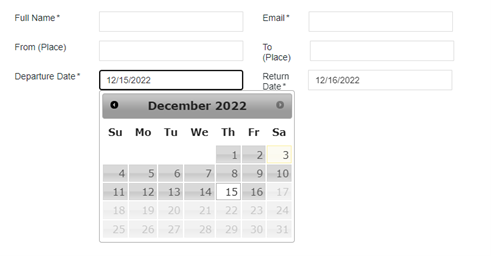
Steps:
- Create a table with two Date/Time type fields for departure and return.
- Create a Submission Form with the required fields.
- For the two Date/Time fields, clear the Calendar popup checkbox.
- Insert Header & Footer.
- In the Header, disable the HTML editor and paste the following code:
- Select the Required checkbox for the relevant fields.
- In the Footer, paste the following code:
- In the pasted code, replace #InsertRecordDepature_Date and #InsertRecordReturn_Date with the actual names of your fields based on this template: #InsertRecord<Field Name>
Learn more about JavaScript elements. - In the last part of the code after function(validator), change the field names of your required fields as well as their related error messages.
The sample code above references four required fields: Full_Name, Email, Departure_Date and Return_Date. If you have more than four required fields, add the script for them using the following template:
Note: This article uses external HTML, JavaScript, or third-party solutions to add functionality outside of Caspio Bridge’s standard feature set. These solutions are provided “as is” without warranty, support, or guarantee. The code within this article is provided as a sample to assist you in the customization of your web applications. You may need a basic understanding of HTML and JavaScript to implement successfully.
For assistance with further customization based on your specific application requirements, please contact our Professional Services team.
code1
<link href="https://code.jquery.com/ui/1.10.4/themes/smoothness/jquery-ui.css" rel="Stylesheet" type="text/css" /> <script type="text/javascript" src="https://code.jquery.com/jquery-1.7.2.min.js"></script> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jqueryui/1.8.11/jquery-ui.min.js"></script> <script src="https://unpkg.com/just-validate@latest/dist/just-validate.production.min.js"></script>
code3
.addField('#InsertRecordField Name', [ { rule: 'required', errorMessage: '! Your error message', }
code2
<script type="text/javascript"> document.addEventListener('DataPageReady', function (event) { $('#InsertRecordDeparture_Date, #InsertRecordReturn_Date').unbind(); $('#InsertRecordDeparture_Date, #InsertRecordReturn_Date').attr('autocomplete', 'off'); init_datepicker(); // Disables numeric keys for inserting date manually $('#InsertRecordDeparture_Date, #InsertRecordReturn_Date').keypress(function(event){ event.preventDefault(); }); // refresh datepicker $('#InsertRecordDeparture_Date, #InsertRecordReturn_Date').keyup(function(event){ console.log(2); init_datepicker(); }); }); function init_datepicker() { let departureDate = $("*[name=InsertRecordDeparture_Date]"); let returnDate = $("*[name=InsertRecordReturn_Date]"); $('.hasDatepicker').removeClass('hasDatepicker'); $('*[name=InsertRecordDeparture_Date], *[name=InsertRecordReturn_Date]').datepicker('destroy'); departureDate.datepicker({ onSelect: function(date){ returnDate.datepicker( "option", "minDate", date); } }); returnDate.datepicker({ onSelect: function(date){ departureDate.datepicker( "option", "maxDate", date); } }); if( departureDate.val() > '' ) { returnDate.datepicker( "option", "minDate", departureDate.val()); } if( returnDate.val() > '' ) { departureDate.datepicker( "option", "maxDate", returnDate.val()); } // remove additional datepicker on datapage reload $('.ui-datepicker').each(function(i){ if (i != 0) { $(this).remove(); } }); } function initCaspioFormValidate(formAppKey, initializer, justValidateConfig = null) { // Make sure the JustValidate is loaded if (typeof window.JustValidate !== 'function') { console.error('JustValidate is not detected. Unable to initialize validator.'); return false; } let validator = null; // Validator instance let formIsValid = false; // Flag to check if the form is already validated. // Use DataPage ready to make sure the form inputs will be detected. document.addEventListener('DataPageReady', function (event) { if (event.detail.appKey.includes(formAppKey)) { if (document.querySelector(`form[action*="${formAppKey}"] section[data-cb-name="cbTable"]`) == null) { // Skip when the form is submitted and the inputs are no longer available. console.log('Form is no longer available. Skipping validation initialization'); return false; } validator = new window.JustValidate(`form[action*="${formAppKey}"]`, justValidateConfig); initializer(validator); } }); // Intercept Caspio form submission so we can validate it first. document.addEventListener('BeforeFormSubmit', function(event) { // A in AppKey is capitalized in BeforeFormSubmit event if (event.detail.AppKey.includes(formAppKey)) { if (!formIsValid) { event.preventDefault(); // Stop form submission validator.revalidate().then(isValid => { formIsValid = isValid; // Update flag so next submit won't validate. if (formIsValid) { // Try to submit again document.querySelector(`form[action*="${formAppKey}"] input[type="submit"]`).click(); } }); } } }); document.addEventListener('FormSubmitted', function(event) { // A in AppKey is capitalized in FormSubmitted event if (event.detail.AppKey.includes(formAppKey)) { formIsValid = false; // Update flag to enable validation again } }); } initCaspioFormValidate( '[@cbAppKey]', function(validator) { validator .addField('#InsertRecordFull_Name', [ { rule: 'required', errorMessage: '! Full Name is required', } ]) .addField('#InsertRecordEmail', [ { rule: 'required', errorMessage: '! Email is required', } ]) .addField('#InsertRecordDeparture_Date', [ { rule: 'required', errorMessage: '! Departure Date is required', } ]) .addField('#InsertRecordReturn_Date', [ { rule: 'required', errorMessage: '! Return Date is required', } ]) .onFail((fields) => { console.log('failed fields: ', fields) }); } ); </script>