Enforcing Options From Autocomplete Fields
3 minutes to read
You can ensure that users enter only specific values in an autocomplete field by using a predefined table with a list of available options. This way, you can prevent the input of custom values, which helps you collect clean and expected data within that field. By enforcing specific values in an autocomplete field, you can enhance data integrity and streamline the user experience by guiding users to select from the predefined set of options rather than allowing the input of unexpected or incorrect values.
Note: This solution supports up to 20,000 values in your lookup list.
Before
After
Steps:
- On your Submission Form DataPage, create and configure an autocomplete form element:
- Select the Required checkbox. If you do not set the field as required and the entered value does not match any value in the source lookup table, a record will be submitted with an empty value.
- In the Lookup table or view list, select the table that contains the values that you want to display in the field, for example, Contacts_Tbl.
- Add and configure a virtual field:
- Leave the Label field empty.
- In the Form element list, select Dropdown.
- In the Source list, select Lookup table or view.
- In the Lookup table section, in the Table or view list, select the same source table as for the autocomplete field, for example, Contacts_Tbl.
- In the Field for display and Field for value lists, select the same source field as in the Filter Autocomplete by setting of the autocomplete field, for example, Company_Name.
- Add a Header & Footer.
- In the footer of the DataPage, disable the HTML editor, and then add the following JavaScript code, changing the values of the
const virtualField
andconst autoComplete
variables to match the corresponding names of virtual and autocomplete fields: - In the header of the DataPage, enter the following code, replacing the number in the
#cbParamVirtual
variable to match the order number of the virtual field if there are more than one such fields: - Optional: If you want to change the text of the error message, you can customize the following code fragment:
The
${HTMLAutocomplete.value}
is the placeholder of the entered value. You can enter it in any place in your code.
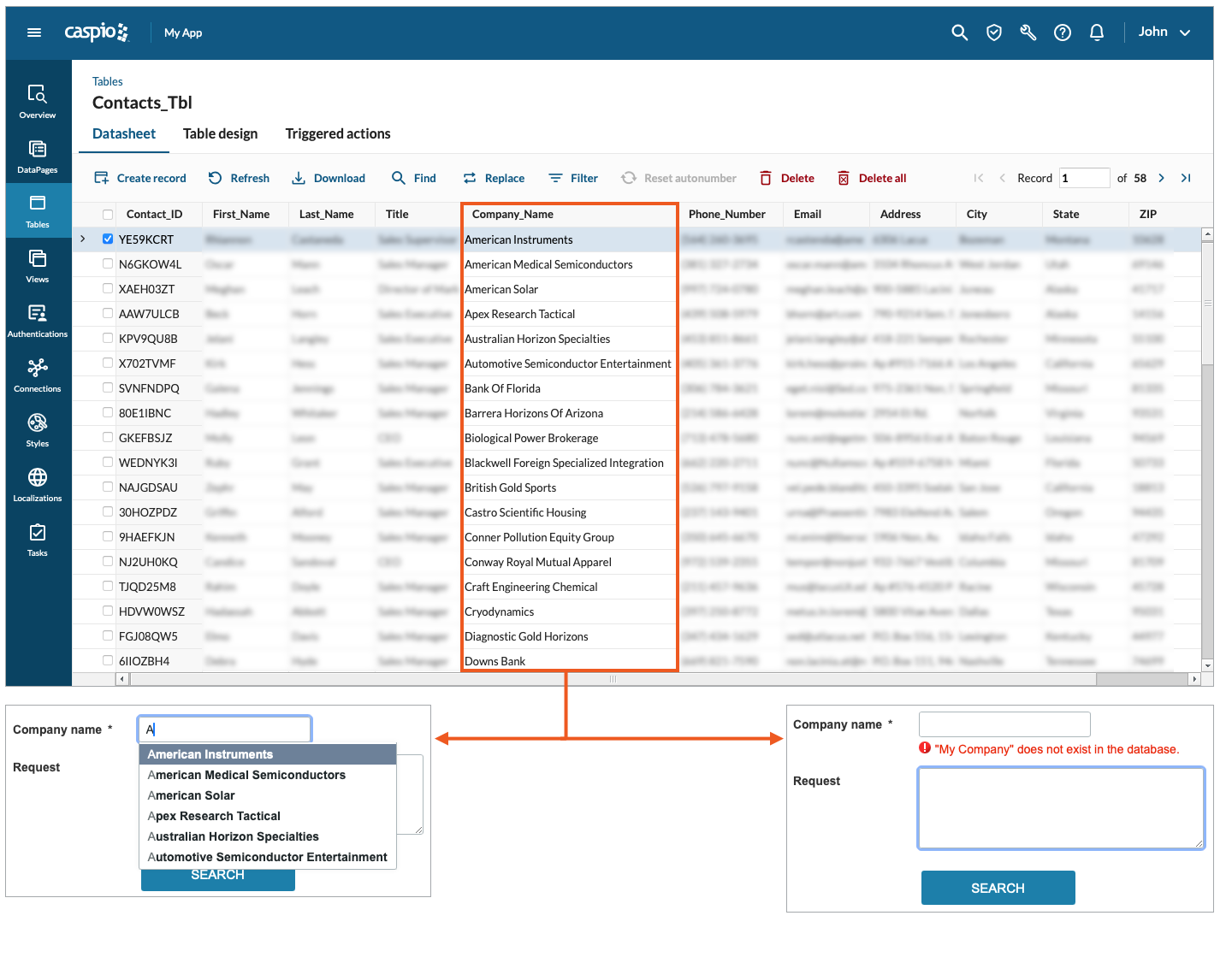
Note: This article uses external HTML, JavaScript, or third-party solutions to add functionality outside of Caspio Bridge’s standard feature set. These solutions are provided “as is” without warranty, support, or guarantee. The code within this article is provided as a sample to assist you in the customization of your web applications. You may need a basic understanding of HTML and JavaScript to implement successfully. For assistance with further customization based on your specific application requirements, please contact our Professional Services team.
code1
<script> if (typeof validateAutocomplete=='undefined') { const virtualField = 'Virtual1' const autoComplete = 'Text_input' const ArrayOfAllowedValues = [] const setArrayOfAllowedValues = () => { document.querySelectorAll(`[id^="cbParam${virtualField}"] option`).forEach(option=>{ ArrayOfAllowedValues.push(option.value.trim()) }) } const validateAutocomplete = (HTMLAutocomplete) => { if(ArrayOfAllowedValues.indexOf(HTMLAutocomplete.value.trim())>-1 || HTMLAutocomplete.value.trim() =='') {return} HTMLAutocomplete.insertAdjacentHTML('afterend', `<span class="cbFormError" id="customErrorMessage"><span class="cbFormErrorMarker"><img src="[@cbBridgeServer]/images/error_icon_exclamation_disc.gif" alt="Exclamation-disc" style="border: 0px;" width="14" height="14"></span> "${HTMLAutocomplete.value} " does not exist in the database.</span>`) HTMLAutocomplete.value = '' } const addListener = () => { let HTMLAutoComplete = document.querySelector(`input[id^="InsertRecord${autoComplete}"]`) HTMLAutoComplete.addEventListener('change', (e)=>{ validateAutocomplete(e.target) }) HTMLAutoComplete.addEventListener('input', (e)=>{ if(document.querySelector('#customErrorMessage')!=null) { document.querySelector('#customErrorMessage').remove() } }) } const DataPageReadyHandler = () => { addListener () setArrayOfAllowedValues() } document.addEventListener('DataPageReady', DataPageReadyHandler ) } </script>
code2
<style> #cbParamVirtual1 { display: none; } </style>
code3
"${HTMLAutocomplete.value}" does not exist in the database.