At times it is necessary to allow your users to input dates in individual fields such as a day, month, year, hour, minute, and second. In Caspio, you can accomplish it with Calculated Value or using JavaScript. This article will guide you on both solutions.
Date Parts:
Part: | Day | / | Month | / | Year | Space | Hour | : | Minute | : | Second |
---|---|---|---|---|---|---|---|---|---|---|---|
Example: | 05 | / | 11 | / | 2011 | 12 | : | 30 | : | 00 |
Note: If the data type of your field is a date/time field, you must include a day / month / year portion even if it is not used later in your app.
Prerequisites:
Ensure that you have a table or a view with at least one Date/Time field.
Steps to separate input fields for Date/Time parts with Calculated Value:
- Edit a Submission Form DataPage.
- In the Select Fields screen, select a Date/Time field and click Next.
- In the Configure Fields screen > General Options, change the Label field to Date and select Calculated Value in the Form element.
- In the Formula field, insert the following formula:
- Add other five virtual fields to collect the following time data:
- Virtual1 - Date
- Virtual2 – Hour
- Virtual3 – Minutes
- Virtual4 – Seconds
- Virtual5 – AM/PM
- Click Insert to add a virtual field.
- In the Field Options section, set the field width to two characters.
- Set the maximum and minimum character length to two characters. This serves as an insurance policy to make sure the input information will be formatted correctly.
- Click the Advanced tab and in the Layout Options section, select Continue next element on same line.
- In the External Associations and Defaults section, select Receive value or parameter.
- Select Default Value from the dropdown and set the value to 00. This is a good visual clue to your users that they need to enter two digits into each of these fields.
- Save a DataPage.
Steps to separate input fields for Date/Time parts with JavaScript:
You can implement a JavaScript to retrieve the date parts and concatenate them into the appropriate format in a DataPage. A sample JavaScript is provided for you to edit in your DataPage.
- Edit an a Submission Form DataPage.
- In the Select Fields screen, select a Date/Time field and click Next.
- In the Configure Fields screen, add a virtual field to collect the Day, Month, and Year date parts.
- Click Insert to add a virtual field.
- In the General Options section, change the Label field to Date.
- In the Field Options section, select Calendar popup. When a user uses the calendar popup tool, the first three date parts are available in the correct format (including slashes).
- Add three more virtual fields to collect the Hour, Minute, and Second date parts. Perform the following steps for each of the fields:
-
- Click Insert to add a virtual field.
- In the Field Options section, set the field width to two characters.
- Set the maximum and minimum character length to two characters. This serves as an insurance policy to make sure the input information will be formatted correctly.
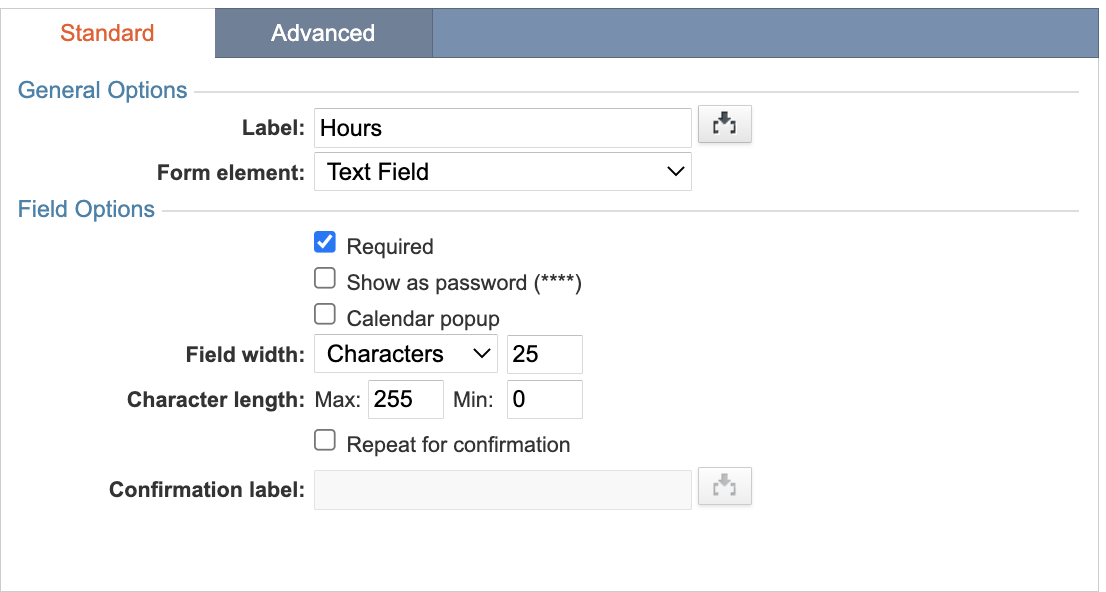
- Click the Advanced tab and in the Layout Options section select Continue next element on same line.
- In the External Associations and Defaults section, select Receive value or parameter.
- Select Default Value from the dropdown and set the value to 00. This is a good visual clue to your users that they need to enter two digits into each of these fields.
- Adjust the labels. The second virtual field (hour) can be labelled Time and the other two virtual fields colon “:”.
- Add a virtual field to handle the AM and PM periods.
- Change the Form element to Dropdown.
- In the Custom Values tab control, add AM and PM. If you use a 24-hour clock localization such as English (UK), change the value of AM to 0 (zero) and PM to 12.
- Add and modify the JavaScript. Using the Insert button add header and footer sections to your DataPage. Then select the Footer section, go to the Source mode and add the following JavaScript.
Note: The JavaScript snippet is different based on the localization that you use. Make sure to use the proper snippet provided below.
English (UK) localization (24-hour clock):
English (US) localization (12-hour clock):
In order for this JavaScript to function correctly, you may need to rename some of the ID names in the preceding JavaScript.
- Virtual1 – Date Input
- Virtual2 – Hour input
- Virtual3 – Minute input
- Virtual4 – Second input
- Virtual5 – AM/PM input
- Date_Field – The “real” table-based input field that can record the data
- Optional: Test your application.
- Click Preview of your DataPage in Caspio. When you hover over the Submit button, your non-virtual, table based-input field (Submitted Date) is populated with the concatenated values from all of the Virtual fields.
- If the field does not populate you might have entered a field ID incorrectly. It can be difficult to find exactly where your error is without a debugging tool such as FireBug. FireBug is a free FireFox plugin that can show you exactly where your JavaScript has an error.
- When the table-based input field populates correctly, you can open the DataPage wizard again and set that field to hidden. In this live example, the field is not hidden so you can see what a functioning JavaScript snippet looks like.
- Be sure to test your form with some sample data after the field has been hidden to be sure it is working correctly.
Note: This article uses external HTML, JavaScript, or third-party solutions to add functionality outside of Caspio standard feature set. These solutions are provided “as is” without any warranty, support, or guarantee. The code within this article is provided as a sample to assist you in the customization of your web applications. You might need a basic understanding of HTML and JavaScript to implement successfully.
For assistance with further customization based on your specific application requirements, please contact our Professional Services team.
code1
<script> document.getElementsByName('Submit')[0].onmouseover = function(){ var date = document.getElementById('cbParamVirtual1').value; if(date == ""){ date = "1/1/2011"; } var hour = parseFloat(document.getElementById('cbParamVirtual2').value) + parseFloat(document.getElementById('cbParamVirtual5').value); if(hour =="24") { hour="12"; } else if(hour =="12") { hour="00"; } var minute = document.getElementById('cbParamVirtual3').value; if(minute == "") { minute = "00"; } var second = document.getElementById('cbParamVirtual4').value; if(second == "") { second = "00"; } document.getElementById('InsertRecordDate_Field').value = date + " " + hour +":"+ minute +":"+second; }; </script>
code2
<script> document.getElementsByName('Submit')[0].onmouseover = function(){ var date = document.getElementById('cbParamVirtual1').value; if(date == "") { date = "1/1/2011"; } var hour = document.getElementById('cbParamVirtual2').value; if(hour =="") { hour="00"; } var minute = document.getElementById('cbParamVirtual3').value; if(minute == "") { minute = "00"; } var second = document.getElementById('cbParamVirtual4').value; if(second == "") { second = "00"; } var ampm = document.getElementById('cbParamVirtual5').value; document.getElementById('InsertRecordActual_DateTime').value = date + " " + hour + ":" + minute + ":" + second + " " + ampm; }; </script>
code3
CASE WHEN '[@cbParamVirtual5]'='AM' OR ( '[@cbParamVirtual5]' = 'PM' AND '[@cbParamVirtual2]' = '12') THEN CONVERT(DATETIME, ('[@cbParamVirtual1]'+' '+'[@cbParamVirtual2]'+':'+'[@cbParamVirtual3]'+':'+'[@cbParamVirtual4]')) WHEN '[@cbParamVirtual5]'='AM' AND '[@cbParamVirtual2]' = '12' THEN CONVERT(DATETIME, ('[@cbParamVirtual1]'+' '+'00'+':'+'[@cbParamVirtual3]'+':'+'[@cbParamVirtual4]')) ELSE CONVERT(DATETIME, ('[@cbParamVirtual1]'+ ' '+CONVERT(VARCHAR,CONVERT(INT,'[@cbParamVirtual2]')+12 )+':'+'[@cbParamVirtual3]'+':'+'[@cbParamVirtual4]')) END