AJAX Loading
3 minutes to readAJAX is a capability that allows immediate communication between Caspio’s servers and deployed DataPages, so users can easily interact with applications without reloading the entire web page. AJAX loading provides a superior user experience and performance of common interactions such as data submission, sorting, paging and refreshing results pages with new criteria.
For applications with multiple DataPages deployed on the same web page, the data will automatically refresh across all DataPages when users interact with the application.
The AJAX loading option was added to the DataPage wizard in Caspio 13.0.
- DataPages created after this version will have AJAX enabled by default.
- DataPages created prior to this version will continue to load without AJAX. We recommend you enable AJAX for existing DataPages, however please see the section below if you are utilizing custom JavaScript or CSS.
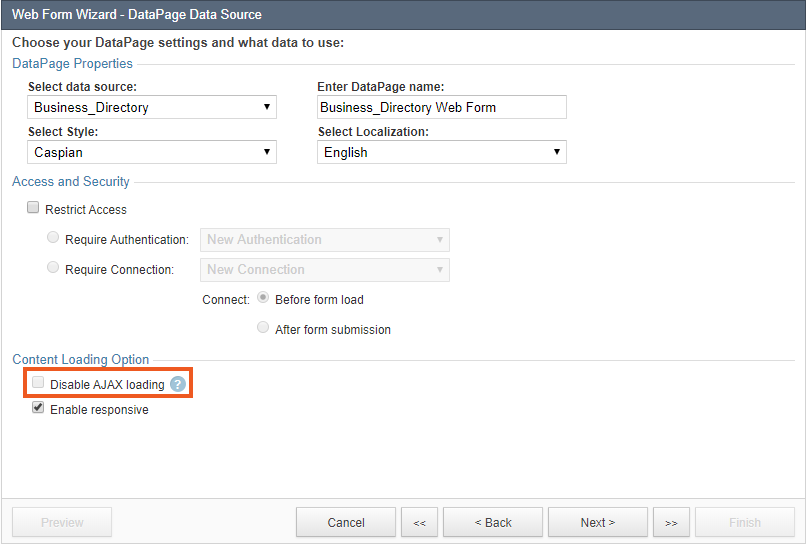
Special Considerations for Custom Scripts
If you are enabling AJAX loading for pre-existing DataPages (created before version 13.0), any custom JavaScript or CSS code inserted into your DataPages must be updated in the following situations:
- document.write() is no longer supported. You will need other methods to output your data (i.e. innerHTML, alert()).
- onload(), onsubmit() and other loading events are no longer supported. You must use the Caspio built-in event handlers, as described below on this page.
- Element ID of buttons in DataPages will be appended with a unique value. For example, the submit button’s ID value will be “Submit_728e87f1676f46” where 728e87f1676f46 is an auto-generated value. You can use a custom parameter to get this unique value, as described below on this page.
Caspio Event Handlers
Caspio provides built-in event handlers to allow you to execute your custom JavaScript/CSS code for events such as adding or deleting records occurs. Similar to JavaScript DOM event listeners, you can use addEventListener(), removeEventListener() and dispatchEvent() to attach, remove or dispatch the Caspio event handlers respectively.
When the event occurs, an event object is passed to the function. The event object structure is described below:
- event.type – the type of event.
- event.detail.appKey – the Appkey of the DataPage in which the event occurs.
- event.detail.uniqueSuffix – the unique ID generated for the DataPage in which the event occurs. This unique ID is appended to the element names in the DataPage.
Available event handlers:
- DataPageReady – this event occurs when DataPages are fully loaded.
- BeforeFormSubmit – this event occurs when a user is attempting to submit a form.
- FormSubmitted – this event occurs after the form is submitted (regardless of whether the submission was successful or returned with an error).
Script Examples
Example 1: A JavaScript code for an event when the DataPages are fully loaded:
<script type="text/javascript"> document.addEventListener('DataPageReady', function (event) { // do something }); </script>
Example 2: A JavaScript code for an event when a specific DataPage is fully loaded. In this example, event.detail.appKey is used to refer to the DataPage through its Appkey:
<script type="text/javascript"> document.addEventListener('DataPageReady', function (event) { if (event.detail.appKey == 'MY_DATAPAGE_APPKEY') { //do something } else if (event.detail.appKey == 'MY_WEB_FORM_APPKEY') { //do something } }); </script>
Example 3: A JavaScript code for an event when a user is attempting to submit a form:
<script type="text/javascript"> document.addEventListener('BeforeFormSubmit', function(event) { // do something }); </script>
Example 4: A Script referring to a DOM element by its ID attribute. In this example, event.detail.appKey and event.detail.uniqueSuffix are used to refer to the DataPage through its Appkey and retrieve the unique ID of the element:
<script type="text/javascript"> document.addEventListener('DataPageReady', function (event) { if (event.detail.appKey == 'MY_DATAPAGE_APPKEY') { //get the unique id for DOM element var elementID = event.detail.uniqueSuffix; var object = document.getElementById('ELEMENT_NAME' + elementID) ; } else if (event.detail.appKey == 'MY_WEB_FORM_APPKEY') { //do something } }); </script>