As an app author, you might want your users to be able to collapse or expand all groups of records on a Tabular Report results page with the “Collapsible group” option enabled. This might be useful, for example, if you expect your users to view all records at once regardless of their grouping.
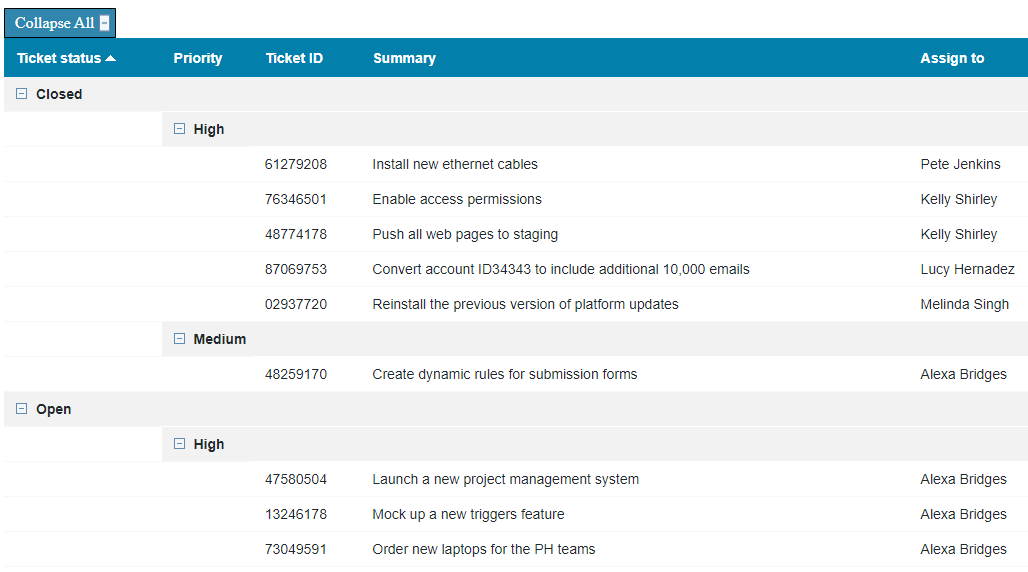
Sample tabular report with expanded groups of records

Sample tabular report with collapsed groups of records
Steps:
- Edit your tabular report. On the Configure Results Page Fields screen, enable data grouping for at least one field.
- From the Grouping display dropdown, select Collapsible group. Using one of the radio buttons below, select if you want the display to be expanded or collapsed by default.
- From the left panel, add Header & Footer.
- In the header, disable the HTML editor and paste the following HTML code:
Result: If the Expanded by default option was selected, a Collapse All button is added.
If Collapsed by default option was selected, the Expand All button is added.
Note: This article uses external HTML, JavaScript, or third-party solutions to add functionality outside of Caspio Bridge’s standard feature set. These solutions are provided “as is” without warranty, support, or guarantee. The code within this article is provided as a sample to assist you in the customization of your web applications. You may need a basic understanding of HTML and JavaScript to implement successfully.
For assistance with further customization based on your specific application requirements, please contact our Professional Services team.
code1
<style> .toggle-button { display: flex; width: 100px; border: 1px solid black; padding: 5px; border-radius: 1px; justify-content: space-between; background: #3186ad; color: white; } .toggle-button:hover { cursor: pointer; } .toggle-checkbox, .expand-Icon { display: none; } .toggle-checkbox:checked ~ .collapse-Icon { display: none; } .toggle-checkbox:checked ~ .expand-Icon { display: inline; } #toggleText { display: block; margin: 0 auto; } </style> <div class="toggle-container" style="display: none;"> <label class="toggle-button"> <span id="toggleText"> </span> <input type="checkbox" class="toggle-checkbox"> <img class="expand-Icon"></img> <img class="collapse-Icon"</img> </label> </div> <script> if(typeof DataPageReadyHandler == 'undefined') { const toggleOptions = (hideOrShow) => { document.querySelectorAll('.cbTableBorderAttribute').forEach(img=> { if (hideOrShow == 'hide') { if (img.getAttribute('alt').indexOf('collapse') > -1) { setToggleText('Expand All') img.click() } } else { if (img.getAttribute('alt').indexOf('expand') > -1) { setToggleText('Collapse All') img.click() } } }) } const setToggleText = (text) => { document.querySelector('#toggleText').innerText = text } const getToggleButtonIconsURL = () => { let defaultToggleIcon = document.querySelector('.cbResultSetShowHideGroupText img') if (defaultToggleIcon == null) return null let defaultToggleIconURL = defaultToggleIcon.getAttribute('src') if(defaultToggleIconURL.indexOf('exp.gif')>-1) {setToggleButtonInitState('expand')} else {setToggleButtonInitState('collapse')} defaultToggleIconURL = defaultToggleIconURL.replace('col.gif', '').replace('exp.gif', '') return { collapsIconURL: `${defaultToggleIconURL}col.gif`, expandIconURL: `${defaultToggleIconURL}exp.gif`} } const setToggleButtonIconsURL = () => { let defaultToggleImgs = getToggleButtonIconsURL() let toggleBtnContainer = document.querySelector('.toggle-container') if (defaultToggleImgs==null) { toggleBtnContainer.style.display = 'none' } else { toggleBtnContainer.style.display = 'block' toggleBtnContainer.querySelector('.expand-Icon').setAttribute('src', defaultToggleImgs.expandIconURL) toggleBtnContainer.querySelector('.collapse-Icon').setAttribute('src', defaultToggleImgs.collapsIconURL) } } const setToggleButtonInitState = (expandOrCollaps) => { let toggleCheckBox = document.querySelector('.toggle-checkbox') if (expandOrCollaps=='expand') { toggleCheckBox.checked = true setToggleText('Expand All') } else { toggleCheckBox.checked = false setToggleText('Collapse All') } } const DataPageReadyHandler = () => { setToggleButtonIconsURL() const toggleCheckbox = document.querySelector('.toggle-button input') toggleCheckbox.addEventListener('change', e => { if (e.target.checked) { toggleOptions('hide') } else { toggleOptions('show') } }) document.removeEventListener('DataPageReady', DataPageReadyHandler) } document.addEventListener('DataPageReady', DataPageReadyHandler) } </script>